Python API Reference¶
BoolExpr is an open source library for symbolic Boolean Algebra.
Data Types:
- abstract syntax tree
A nested tuple of entries that represents an expression. Unlike a
BoolExpr
object, an ast object is serializable.It is defined recursively:
ast := (BoolExpr.Kind.zero, ) | (BoolExpr.Kind.one, ) | (BoolExpr.Kind.log, ) | (BoolExpr.Kind.comp, ctx, name) | (BoolExpr.Kind.var, ctx, name) | (BoolExpr.Kind.nor, ast, ...) | (BoolExpr.Kind.or_, ast, ...) | (BoolExpr.Kind.nand, ast, ...) | (BoolExpr.Kind.and_, ast, ...) | (BoolExpr.Kind.xnor, ast, ...) | (BoolExpr.Kind.xor, ast, ...) | (BoolExpr.Kind.neq, ast, ...) | (BoolExpr.Kind.eq, ast, ...) | (BoolExpr.Kind.nimpl, ast, ast) | (BoolExpr.Kind.impl, ast, ast) | (BoolExpr.Kind.nite, ast, ast, ast) | (BoolExpr.Kind.ite, ast, ast, ast) ctx := int name := str
The
ctx
int is a pointer to a C++ Context object. It must be re-cast tovoid *
before being used.- point
- A dictionary of
{Variable : Constant}
mappings. For example,{a: False, b: True, c: 0, d: 'X'}
. - var2bx
- A dictionary of
{Variable : BoolExpr}
mappings. For example,{a: False, b: ~p | q}
.
Symbolic Variable Context¶
-
class
boolexpr.
Context
[source]¶ A context for Boolean variables
-
get_vars
(name, *dims)[source]¶ Return a multi-dimensional array of variables.
The name argument is a
str
.The variadic dims input is a sequence of dimension specs. A dimension spec is a two-tuple: (start index, stop index). If a dimension is given as a single
int
, it will be converted to(0, stop)
.The dimension starts at index
start
, and increments by one up to, but not including,stop
. This follows the Python slice convention.
-
Boolean Expression Class Hierarchy¶
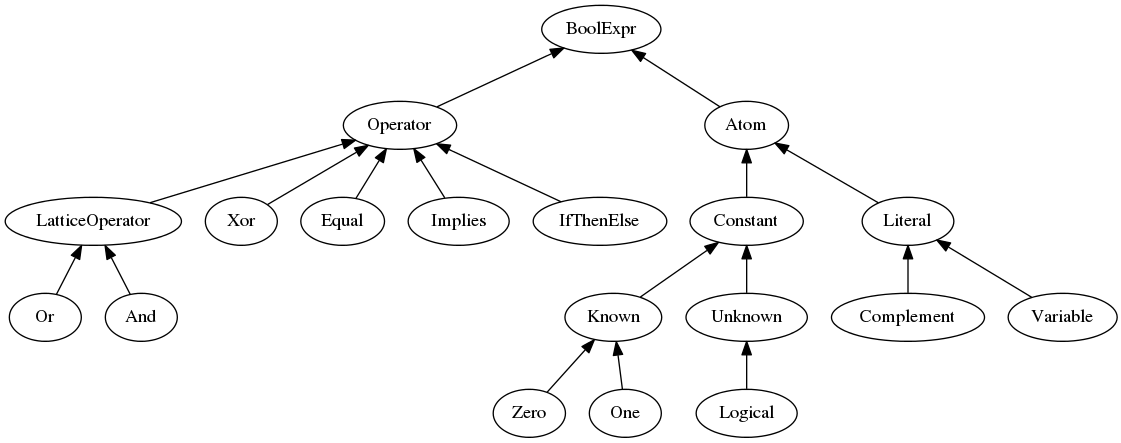
Note
For the sake of space, this diagram omits the
Nor
, Nand
, Xnor
, Unequal
, NotImplies
,
and NotIfThenElse
classes.
They are derived from Operator
.
Base Class¶
-
class
boolexpr.
BoolExpr
(cdata)[source]¶ Wrap boolexpr::BoolExpr class
-
BoolExpr.
__or__
(other)[source]¶ Boolean disjunction (sum, OR) operator
\(f\) \(g\) \(f + g\) 0 0 0 0 1 1 1 0 1 1 1 1
-
BoolExpr.
__and__
(other)[source]¶ Boolean conjunction (product, AND) operator
\(f\) \(g\) \(f \cdot g\) 0 0 0 0 1 0 1 0 0 1 1 1
-
BoolExpr.
__xor__
(other)[source]¶ Boolean exclusive or (XOR) operator
\(f\) \(g\) \(f \oplus g\) 0 0 0 0 1 1 1 0 1 1 1 0
-
BoolExpr.
kind
¶ Return the Kind code.
The expression “kind” is one byte of information. See
include/boolexpr/boolexpr.h
for the full table of kinds codes. For convenience, this value is wrapped by a Python Enum.
-
BoolExpr.
depth
()[source]¶ Return the depth of the expression.
- An
Atom
has zero depth. - An
Operator
has depth equal to the maximum depth of its arguments plus one.
- An
-
BoolExpr.
size
()[source]¶ Return the size of the expression.
- An
Atom
has size one. - An
Operator
has size equal to the sum of its arguments’ sizes plus one.
- An
-
BoolExpr.
is_cnf
()[source]¶ Return
True
if the expression is in conjunctive normal form (CNF).A CNF is defined as a conjunction of clauses, where a clause is defined as either a
Literal
, or a disjunction of literals.Boolean True (
And
identity) is also considered to be a CNF.
-
BoolExpr.
is_dnf
()[source]¶ Return
True
if the expression is in disjunctive normal form (DNF).A DNF is defined as a disjunction of clauses, where a clause is defined as either a
Literal
, or a disjunction of literals.Boolean False (
Or
identity) is also considered to be a DNF.
-
BoolExpr.
simplify
()[source]¶ Return a simplified expression.
Simplification uses Boolean algebra identities to reduce the size of the expression.
-
BoolExpr.
to_posop
()[source]¶ Return an expression with NOT bubbles pushed down through dual ops.
Specifically, perform the following transformations:
\[ \begin{align}\begin{aligned}\overline{a + b + \ldots} &\iff \overline{a\vphantom{b}} \cdot \overline{b} \cdot \ldots\\\overline{a \cdot b \cdot \ldots} &\iff \overline{a\vphantom{b}} + \overline{b} + \ldots\\\overline{a \oplus b \oplus \ldots} &\iff \overline{a} \oplus b \oplus \ldots\\\overline{eq(a, b, \ldots)} &\iff eq(\overline{a}, b, \ldots)\\\overline{p \implies q} &\iff p \cdot \overline{q}\\\overline{ite(s, d1, d0)} &\iff ite(s, \overline{d1}, \overline{d0})\end{aligned}\end{align} \]
-
BoolExpr.
tseytin
(ctx, auxvarname='a')[source]¶ Return the Tseytin transformation.
The
ctx
parameter is aContext
object that will be used to store auxiliary variables.The
auxvarname
parameter is the prefix of auxiliary variable names. The suffix will be in the form_0
,_1
, etc.
-
BoolExpr.
compose
(var2bx)[source]¶ Substitute a subset of support variables with other Boolean expressions.
Returns a new expression: \(f(g_i, \ldots)\)
\(f_1 \: | \: x_i = f_2\)
-
BoolExpr.
restrict
(point)[source]¶ Restrict a subset of support variables to \(\{0, 1\}\).
Returns a new function: \(f(x_i, \ldots)\)
\(f \: | \: x_i = b\)
-
BoolExpr.
sat
()[source]¶ Return a tuple (sat, point).
The sat value is
True
if the expression is satisfiable. If the expression is not satisfiable, the point will beNone
. Otherwise, it will return a satisfying input point.
-
BoolExpr.
to_nnf
()[source]¶ Convert the expression to negation normal form (NNF).
Negation formal form is defined as:
- Only
Or
orAnd
operators - All NOT bubbles are pushed down to the literals
- Simple
- Only
-
BoolExpr.
equiv
(other)[source]¶ Return True if the two expressions are formally equivalent.
Note
While in practice this check can be quite fast, SAT is an NP-complete problem, so some inputs will require exponential runtime.
-
BoolExpr.
degree
()[source]¶ Return the degree of a function.
A function from \(B^{N} \Rightarrow B\) is called a Boolean function of degree \(N\).
-
BoolExpr.
smoothing
(xs)[source]¶ Return the smoothing over a sequence of N variables.
The xs argument is a sequence of \(N\) Boolean variables.
The smoothing of \(f(x_1, x_2, \dots, x_i, \dots, x_n)\) with respect to variable \(x_i\) is: \(S_{x_i}(f) = f_{x_i} + f_{x_i'}\)
This is the same as the existential quantification operator: \(\exists \{x_1, x_2, \dots\} \: f\)
-
BoolExpr.
consensus
(xs)[source]¶ Return the consensus over a sequence of N variables.
The xs argument is a sequence of \(N\) Boolean variables.
The consensus of \(f(x_1, x_2, \dots, x_i, \dots, x_n)\) with respect to variable \(x_i\) is: \(C_{x_i}(f) = f_{x_i} \cdot f_{x_i'}\)
This is the same as the universal quantification operator: \(\forall \{x_1, x_2, \dots\} \: f\)
-
BoolExpr.
derivative
(xs)[source]¶ Return the derivative over a sequence of N variables.
The xs argument is a sequence of \(N\) Boolean variables.
The derivative of \(f(x_1, x_2, \dots, x_i, \dots, x_n)\) with respect to variable \(x_i\) is: \(\frac{\partial}{\partial x_i} f = f_{x_i} \oplus f_{x_i'}\)
This is also known as the Boolean difference.
-
Atom Nodes¶
Operator Nodes¶
-
class
boolexpr.
LatticeOperator
(cdata)[source]¶ Bases:
boolexpr.wrap.Operator
Boolean Lattice Operator
-
class
boolexpr.
Unequal
(cdata)[source]¶ Bases:
boolexpr.wrap.NegativeOperator
Boolean Unequal Operator
-
class
boolexpr.
NotImplies
(cdata)[source]¶ Bases:
boolexpr.wrap.NegativeOperator
Boolean Not Implies Operator
Constant Singletons¶
-
boolexpr.
ZERO
= 0¶ Boolean Zero
-
boolexpr.
ONE
= 1¶ Boolean One
-
boolexpr.
LOGICAL
= X¶ Boolean Logical Unknown
-
boolexpr.
ILLOGICAL
= ?¶ Boolean Illogical Unknown
Operator Functions¶
Fundamental Operators¶
Simplifying Operators¶
High Order Operators¶
-
boolexpr.
onehot0
(*args)[source]¶ Return an expression that means “at most one input function is true”.
-
boolexpr.
onehot
(*args)[source]¶ Return an expression that means “exactly one input function is true”.
-
boolexpr.
nhot
(n, *args)[source]¶ Return a CNF expression that means “exactly N input functions are true”.
-
boolexpr.
majority
(*args)[source]¶ Return a CNF expression that means “the majority of input functions are true”.
-
boolexpr.
achilles_heel
(*args)[source]¶ Return the Achille’s Heel function, defined as: \(\prod_{i=0}^{n/2-1}{X_{2i} + X_{2i+1}}\).
-
boolexpr.
mux
(a, sel)[source]¶ Return an expression that multiplexes an input array over a select array.
Multidimensional Arrays¶
-
boolexpr.
zeros
(*dims)[source]¶ Return a multi-dimensional array of zeros.
The variadic dims input is a sequence of dimension specs. A dimension spec is a two-tuple: (start index, stop index). If a dimension is given as a single
int
, it will be converted to(0, stop)
.The dimension starts at index
start
, and increments by one up to, but not including,stop
. This follows the Python slice convention.For example, to create a 4x4 array of zeros:
>>> zeros(2, 4) array([[0, 0, 0, 0], [0, 0, 0, 0]])
-
boolexpr.
ones
(*dims)[source]¶ Return a multi-dimensional array of ones.
The variadic dims input is a sequence of dimension specs. A dimension spec is a two-tuple: (start index, stop index). If a dimension is given as a single
int
, it will be converted to(0, stop)
.The dimension starts at index
start
, and increments by one up to, but not including,stop
. This follows the Python slice convention.For example, to create a 4x4 array of ones:
>>> ones(2, 4) array([[1, 1, 1, 1], [1, 1, 1, 1]])
-
boolexpr.
logicals
(*dims)[source]¶ Return a multi-dimensional array of X’s.
The variadic dims input is a sequence of dimension specs. A dimension spec is a two-tuple: (start index, stop index). If a dimension is given as a single
int
, it will be converted to(0, stop)
.The dimension starts at index
start
, and increments by one up to, but not including,stop
. This follows the Python slice convention.For example, to create a 4x4 array of X’s:
>>> logicals(2, 4) array([[X, X, X, X], [X, X, X, X]])
-
boolexpr.
uint2nda
(num, length=None)[source]¶ Convert unsigned num to an array of expressions.
The num argument is a non-negative integer.
If no length parameter is given, the return value will have the minimal required length. Otherwise, the return value will be zero-extended to match length.
For example, to convert the byte 42 (binary
0b00101010
):>>> uint2nda(42, 8) array([0, 1, 0, 1, 0, 1, 0, 0])
-
boolexpr.
int2nda
(num, length=None)[source]¶ Convert num to an array of expressions.
The num argument is an
int
. Negative numbers will be converted using twos-complement notation.If no length parameter is given, the return value will have the minimal required length. Otherwise, the return value will be sign-extended to match length.
For example, to convert the bytes 42 (binary
0b00101010
), and -42 (binary0b11010110
):>>> int2nda(42, 8) array([0, 1, 0, 1, 0, 1, 0, 0]) >>> int2nda(-42, 8) array([0, 1, 1, 0, 1, 0, 1, 1])
-
boolexpr.
array
(objs, shape=None)[source]¶ Return an N-dimensional array of Boolean expressions.
The objs argument is a nested sequence of homogeneous Boolean expressions. That is, both [a, b, c, d] and [[a, b], [c, d]] are valid inputs.
The optional shape parameter is a tuple of dimension specs, which are
(int, int)
tuples. It must match the volume of objs. The shape can always be automatically determined from objs, but you can supply it to automatically reshape a flat input.
-
class
boolexpr.
ndarray
(bxa, shape=None)[source]¶ N-dimensional array of Boolean expressions
-
shape
¶ Return the Array shape.
-
ndim
¶ Return the number of dimensions.
-
size
¶ Return the size of the array.
The size of a multi-dimensional array is the product of the sizes of its dimensions.
-
flat
¶ Return a 1D iterator over the array.
-